UDP exercises
- Write a program that reads a single line from the standard input, sends a datagram that contains the line to a server and then receives a single datagram from the server.
- Write an eacho-server: the server takes one datagram that contains a single line X and sends back to the client one datagram that contains the line "echo: X".
UDP sockets
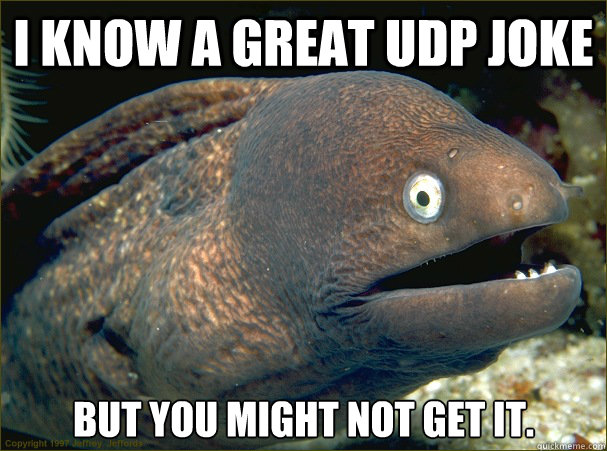
UDP sockets in Java (both unicast and multicast) are represented by class DatagramSocket.
A typical scenario for a UDP server is as follows:
- setting up a datagram socekt on a known port:
server = new DatagramSocket();
- preparing a fresh instance of a datagram (to be filled in with received data)
buff = new byte[MAX_DATAGRAM_SIZE]; datagram = new DatagramPacket(buff, buff.length);
- awaiting for data:
server.recive(datagram);
- creating a thread to communicate with the client:
new Thread() { //... public void run() { // communication with the client //... } }.start();
- in the prologue to the communication - gathering information about client's IP address and port:
clientAddress = datagram.getAddress(); clientPort = datagram.getPort();
Some useful files:
Datagram size: UDP.java |
Simple UDP client: UDPClient.java |
Simple UDP server: UDPServer.java, Factorial.java |
Portmapper TCP
A service is a TCP server (running in the network on a given IP address and a given TCP port number) whose communication protocol is as follows:
- it accepts exactly one line from a client
- responds with exactly one line
REGISTER name ip port
where "name" is a name (a sequence of non-white characters) under which the service running on IP address "ip" and port number "port" has to be registered. After successful registration of the service, protmapper should respond to the client with one line of the form: "Service IP port registered as name". In case the registration is not possible, portmapper should respond with a single line describing the error.GET name
where "name" is a name of a previously registered service. Portmapper should respond on this command with a single line of the form: "ip port", where "ip" is the IP address and "port" is the port number of the service registered under name "name"CALL name args...
where "name" is a name of a previously registered service. After receiving this command the portmapper should establish a connection with the service registered under name "name", send it a single line "args..." (i.e. the rest of the line after "CALL name"), receive a single line from the service and send this line back to the client.
TCP exercises
- Write a program that reads two lines from the standard input, sends them to a server and then reads one line fron the server.
- Write an eacho-server: the server reads two lines X, Y from a client and then sends back to the client one line of the form "echo: X, Y".
- Warite a TCP port scanner
TCP sockets
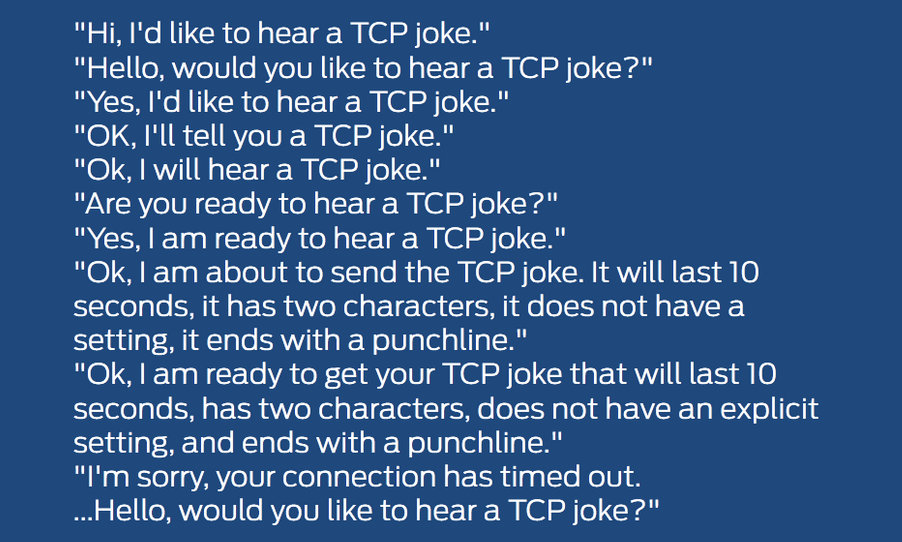
There are two types of TCP sockets:
- listening sockets - used by server applications to await for connections from clients; such sockets are uniquely determined by the address of the host and the port number.
- connection sockets - used by both clients and servers to exchange data; such sockets are uniquely determined by a quadruple: local host, local port, remote host, remote port
Here is a typical structure of a server application:
- setting up a listening socket on a known port (number 0 indicates that the operating system can choose any port number):
server = new ServerSocket(0);
- awaiting for a client
- acceptance of the client (handshake) - as a result we get a connection socket to communicate with the client:
client = server.accept();
- creating a thread to communicate with the client:
new Thread() { //... public void run() { // communication with the client //... } }.start();
- setting up a communication socket and binding it with a remote server socket:
client = new Socket(serverAddress, serverPort);
- communication with the server
Introduction to HTTP protocol
Warriors of the Net
Film "Warriors of the Net" is available through the following site: www.warriorsofthe.net